Libraries and Functions – Fundamentals
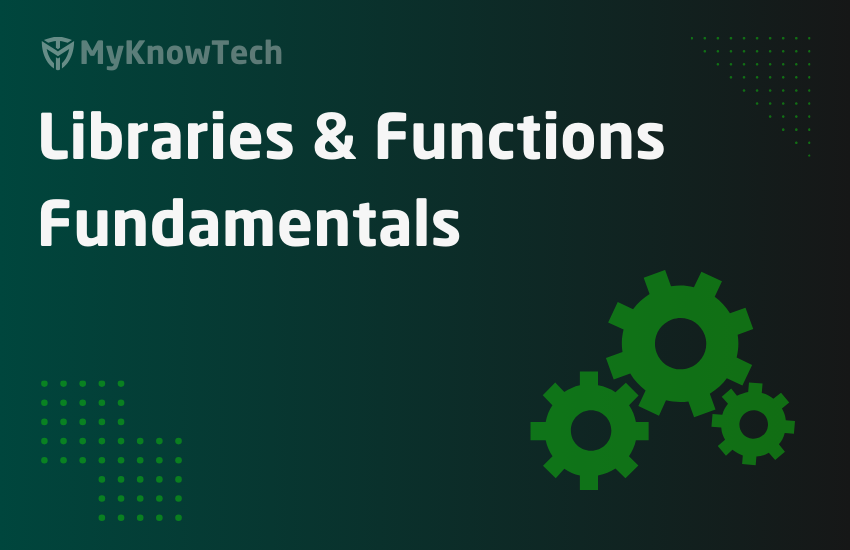
In this blog article, we will talk about the basics of functions and libraries in pega.
Functions in programming language
– Function is a block of reusable code that is used to perform a single related operation/action.
– Functions usually accept input data, process the data and then it may return a result.
– Different programming language name function differently – function, methods, procedures. Sub-routines.
– Many programming languages have their own built in functions in their libraries. You can always define custom functions based on the language.
Example built in functions:
– In Java we have the inbuilt math functions (methods) – length(), sqrt(), equals().. etc
– In Javascript we have functions like toString(), valueOf()…etc
– In python we have – bool(), bin(), len()..etc
Library in Programming language
Collection of pre-written code that we can use to optimize tasks.
Let’s have a look at the Java API library.
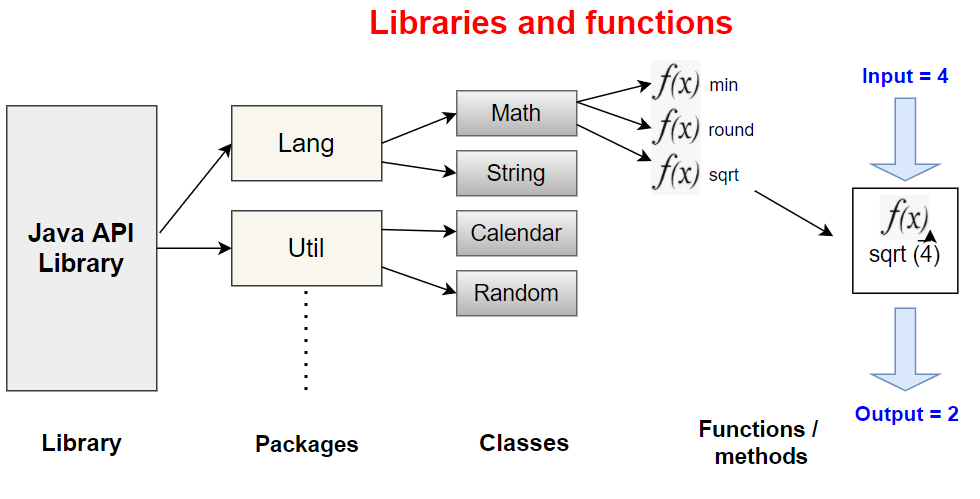
Visit the Java 7 API library documentation – https://docs.oracle.com/javase/7/docs/api/
You will find the list of packages, classes and methods as shown in the below hierarchy.
Library -> Packages -> Classes -> Methods.
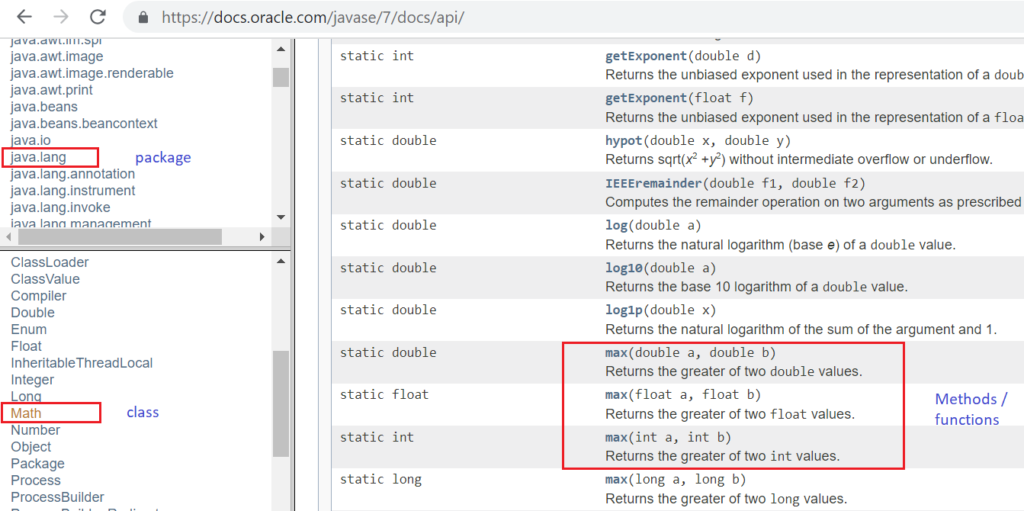
These are all in-built Java functions.
As we know, Pega is a Java-based business process management tool and requires a Java Runtime Environment (JRE). Can our Pega application access all these inbuilt Java methods?? The answer is YES, but how? We will find the answer a little later 😉
Like the Java API documentation, Pega has its own Engine API documentation.
Click on the resources icon and then click the Engine API.
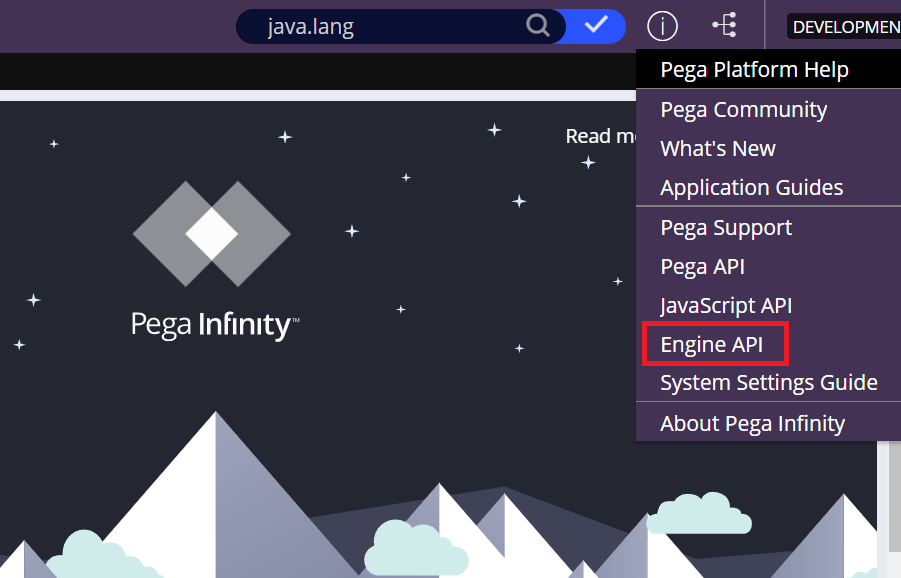
You find all the Java packages, classes and methods that are shipped with the Pega product. These are built in by the Pegasystems and shipped with the pega engine code. Most of the backend engine processes make use of these APIs for the processes.
https://community.pega.com/sites/default/files/help_v87/javadocs/index.html
Tip: As you see the above html page is hosted on the community pega site, instead of navigating from pega application, it can be directly visited in a browser with the above link.
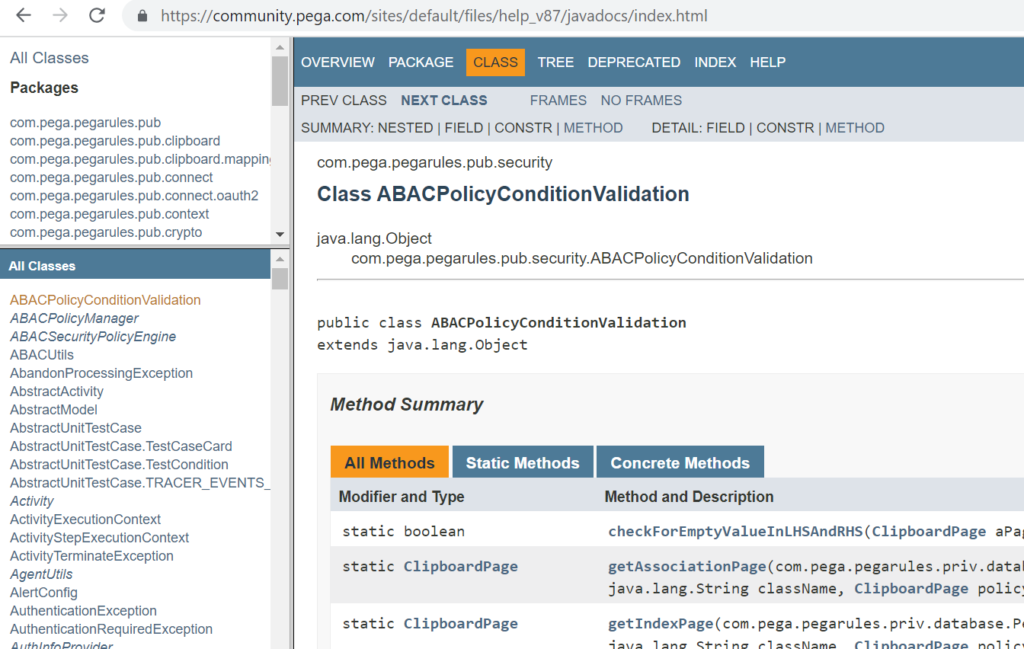
In the Engine API documentation, you find only inbuilt pega engine classes and functions. When you install pega software, all these engine classes with be installed within the Pega database.
Let’s look at a simple example.
For the package – com.pega.pegarules.pub.connect.oauth2 we have a single interface.
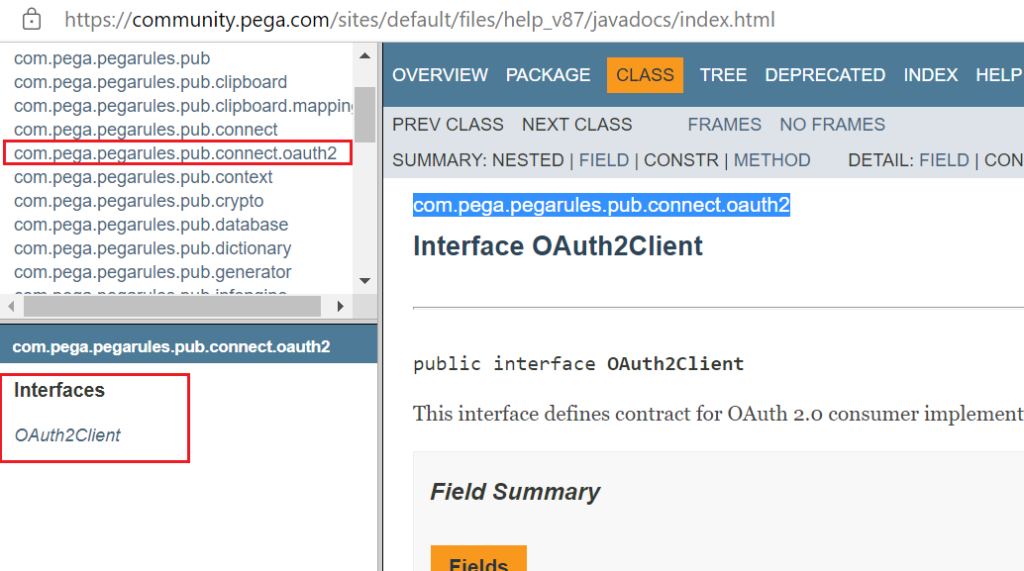
You will also find a single entry in the pega database table in the rule schema – pr_engineclasses
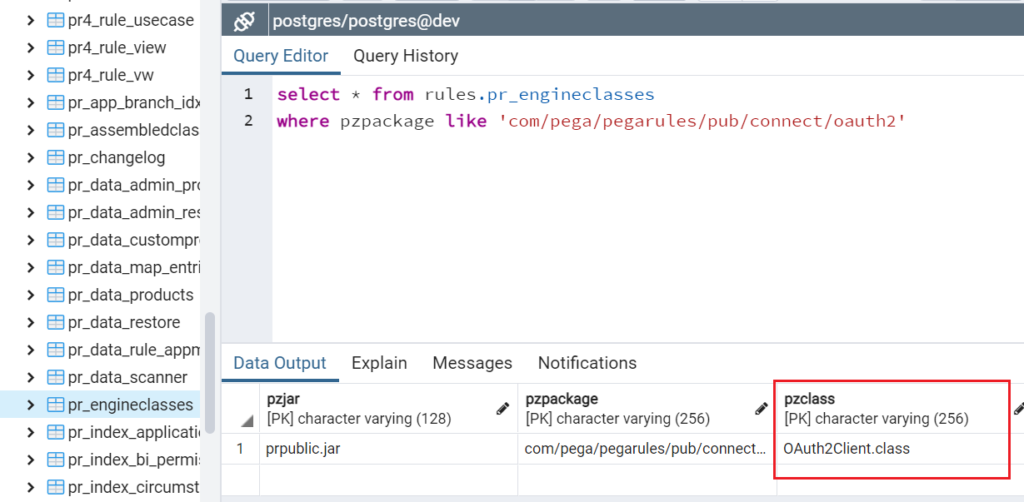
On querying, replace the dots in the package name with the forward-slash (/)
Hence it is proved that all the pega engine classes (which you see in the API documentation) are installed in the pega database.
Now the question is – Are we restricted to using only the engine APIs? Can’t we use any custom Java functions? – Yes we can.
Pega rulebase has two rule categories – Library and Functions as part of the technical category.
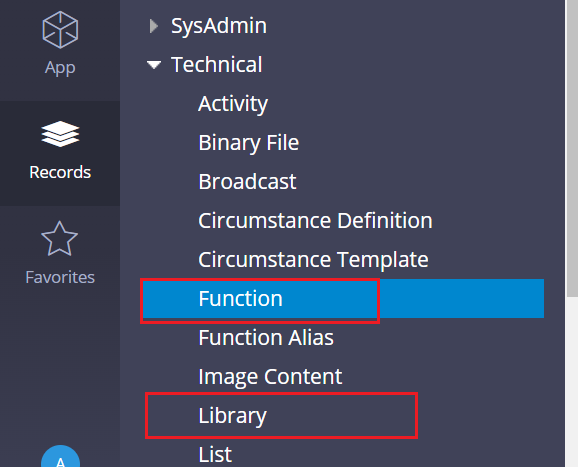
Let’s look at it one by one.
What is a Library instance in Pega?
The main role of a library instance is to hold or group a set of related functions.
Library rule is of technical category and is of class Rule-Utility-Library. Though the library is a rule (Rule-) instance, we cannot lock or checkout the rule. We also cannot change the availability of the library rule. It will always be available.
Pega product OOTB shipped with a rich set of standard libraries and standard functions.
You can view the OOTB libraries Records -> Technical -> Library
You can filter on the library name – Math
Ha ha.. I find Kerim – the Chief Product Officer of Pegasystems. Maybe he was a developer for this rule instance long back 😀

Click and open the instance
What are the configuration points in the Library rule?
There are two tabs
Packages tab
You find the 2 Java packages included in the library.
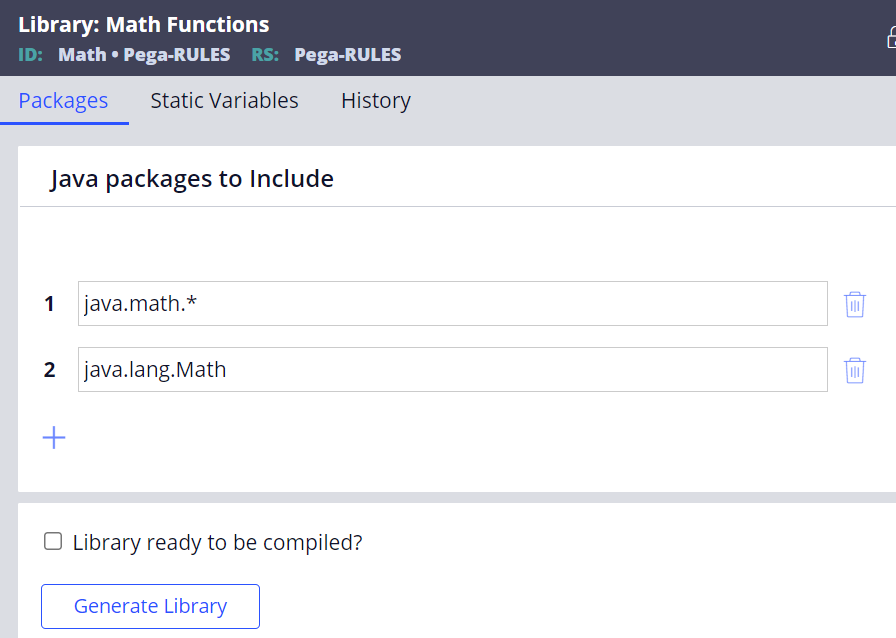
Why do we need to include or import the Java packages?
In Java programming, we can import the necessary packages to make use of the functions/methods inside the package.
For example – In my Java program I need to use nextLine() function that is part of scanner class, then my below java code is like this – I import the package and refer to the function inside the package.
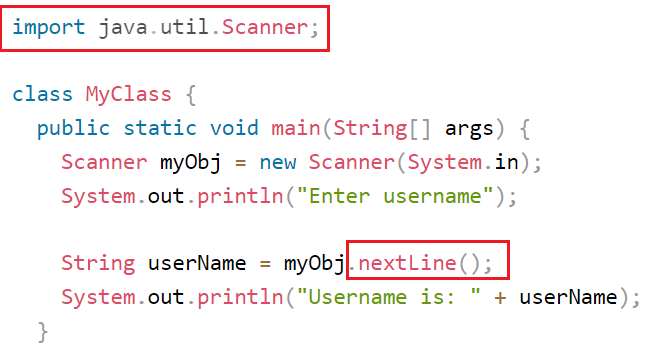
Similarly in Pega, to use the right Math functions, I need to include the right package or class.
Java. math and java.lang.Math is two Java inbuilt packages that contain all the necessary math operations or functions. So we included them in our pega library instance.
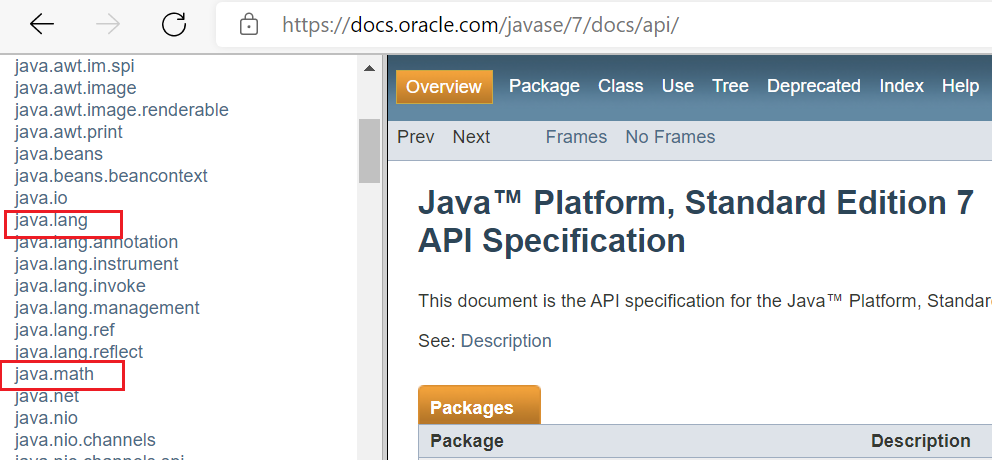
Here we referred to all the classes that are part of java.math package with a wild character (*) and for Java lang, we referred to the class name – Math directly. <packagename>.<classname>
In the rule form, you see an option to generate la ibrary. We will see it together with functions rule exploration.
Static variables tab
You can define the Java static variables or the constants that can be used across all the functions that are packed as part of this library.
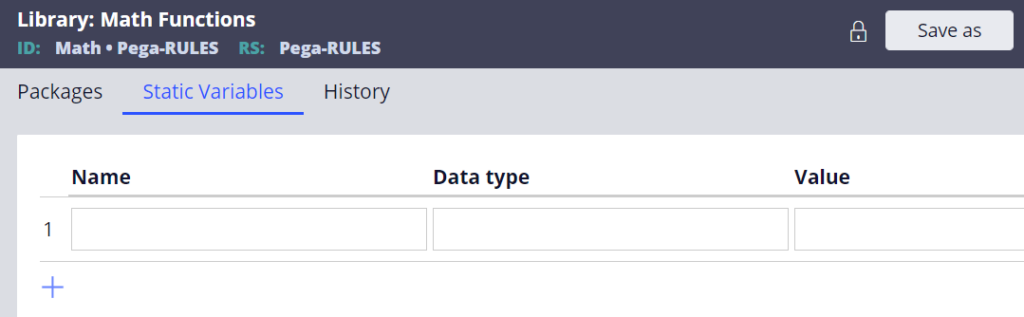
When we do need to create a new library?
We saw Pega ships lot of inbuilt standard libraries and functions. Only when needed, you can go for creating a new library to include the newly created functions.
Tip: Mostly to create custom functions for a Pega application, we end up creating custom library.
Let’s look at the standard functions that are shipped within Math library
Records -> Technical –> Functions and filter on library name = math
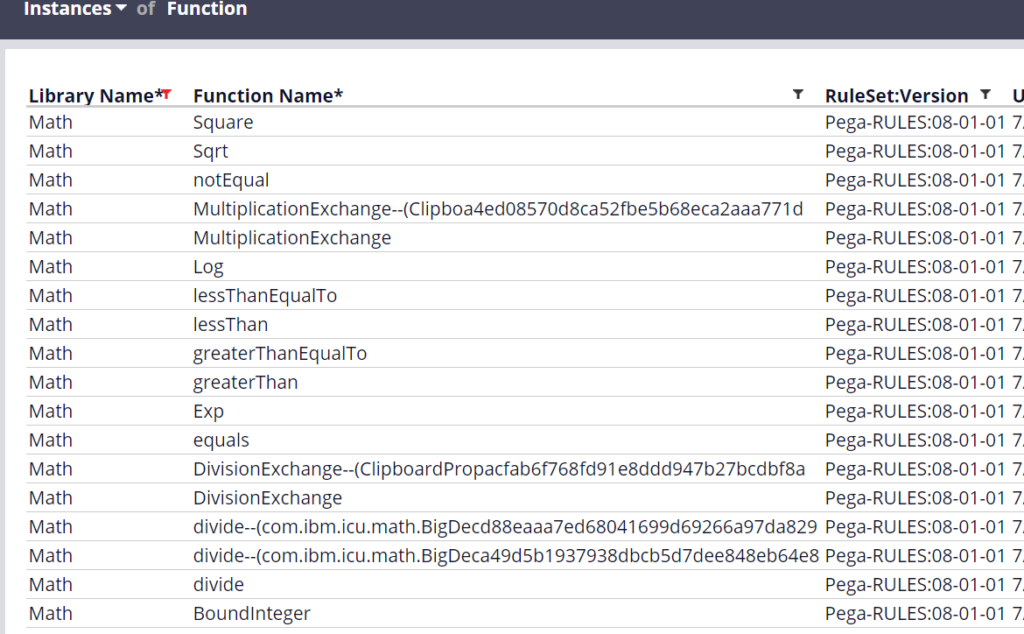
You see there are around 18 functions that are part of library math.
Can you recognize the same functions are part of the Java API library documentation. You see function sqrt() is available in both Pega inbuilt and Java 7 inbuilt functions.
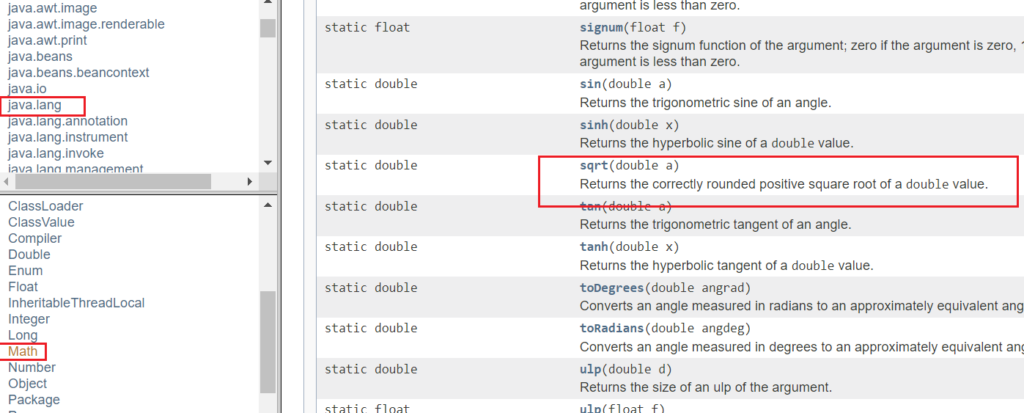
But in the inbuilt Java API documentation there are many more functions that are part of Java.Lang.Math which are not created as Pega function rules.
We saw before Pega applications can access the inbuilt Java API functions, so why do we need to create Pega function rule for the same operations? Does it look redundant?
What is a function rule?
– Function rule defines the input, and output parameters and a Java code that is invoked when calling function.
– It is of technical category and is of class Rule-Utility-Function
– Pega function rules are accessed through rule resolution. It means you can have different versions of function rules at different rulesets and it can be rule resolved based on the application ruleset list.
– Functions can be referenced using the expression builder, activity method call and also via Java step.
The Java API functions can be accessed only via Java code and not via expression builders!! Now you know why Pega created the Pega function rules for the most widely used Java 7 functions. Also one of the main Pega guardrails is to avoid Java coding!!
Click and view the sqrt function rule.
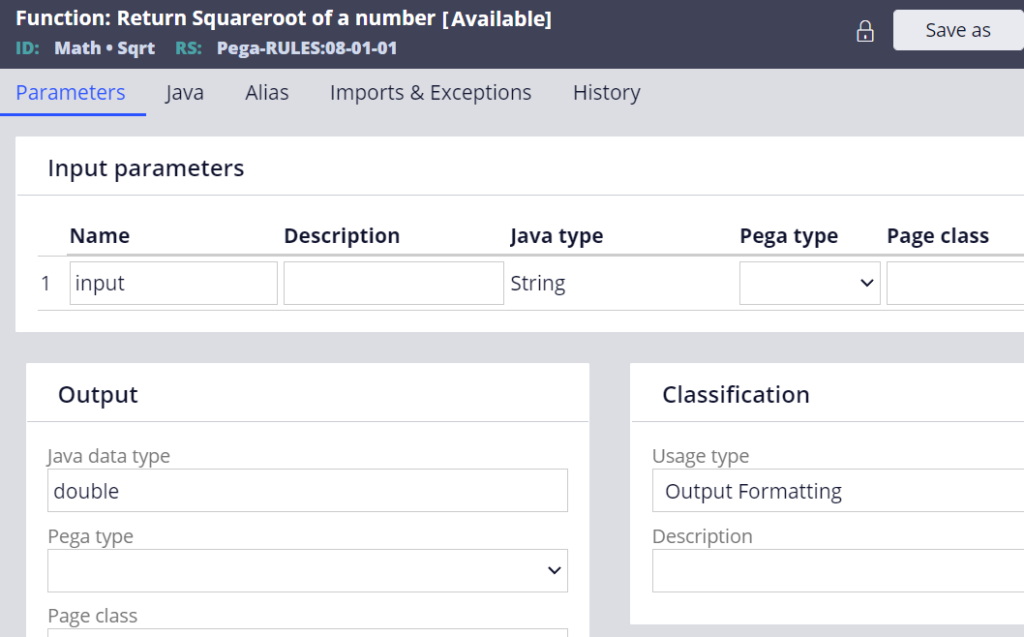
You see the Identifier of the function name – It is always the Library name. function name. This is how you link the function name with the library name on creation.
There are 4 main tabs.
Parameters tab
It accepts the input parameter as string Java type and outputs the Java data type as double.
Note: Pega type and page class are optional for both input and output
Java tab
Click on the java tab, there will be the function processing.
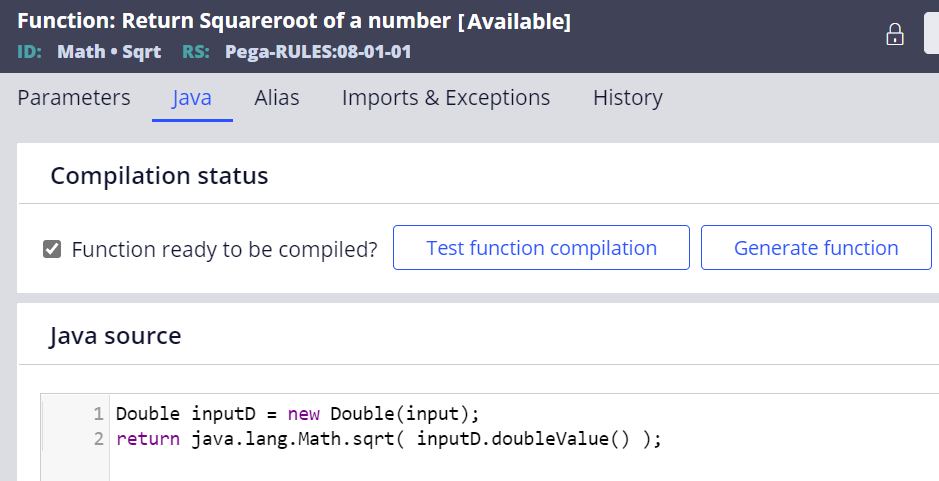
They convert the data type to double and then call the Java API java.lang.Math.sqrt function 😊
At the end, it looks like a wrapper for the inbuilt Java API function to use it Pega application development.
We already know the answer that from a Pega application we can access the inbuilt Java code, but how?
All the inbuilt Java classes are loaded from the JRE lib folder.
In the JRE lib folder, there will be a jar – rt.jar
Rt stands for run time.
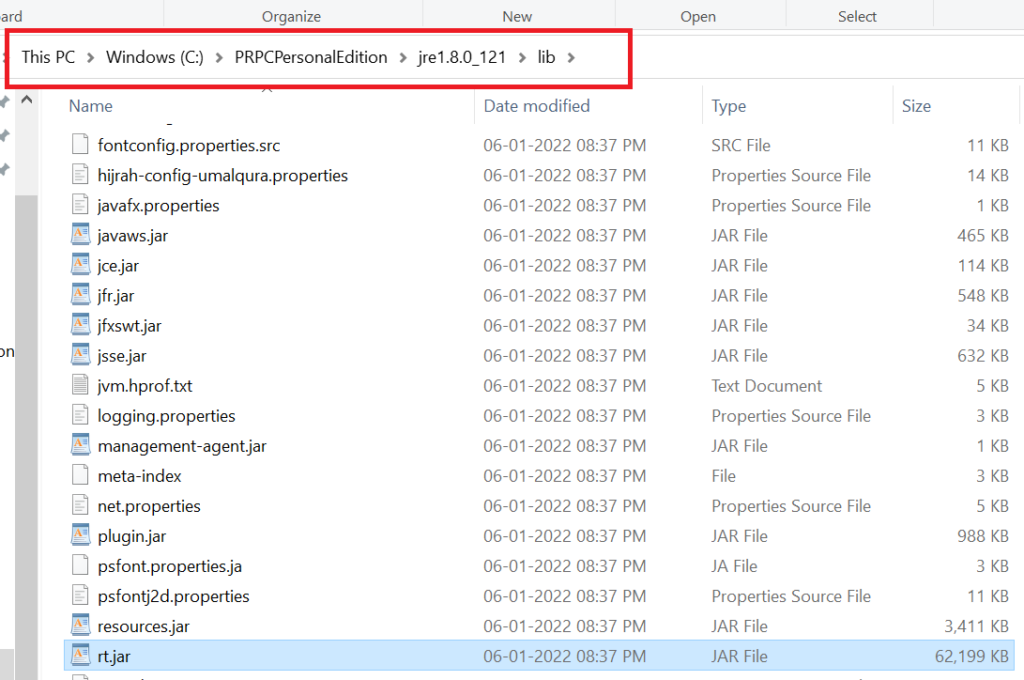
To explore the content, extract the jar and place the files in a separate folder to view the contents.
You can find the java.lang.Math class file in the right folder.
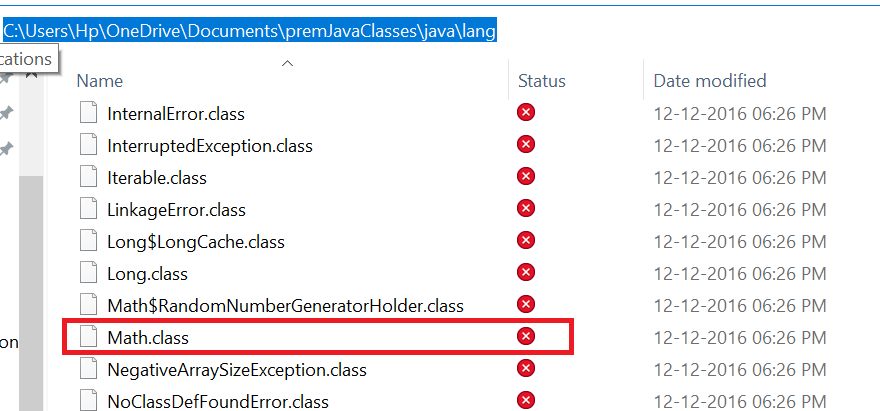
In this way, we can directly access those inbuilt Java 7 classes.
In the library rule form, look at the compilation status block.

We saw a similar Generate Library configuration in the Library rule form.
Function ready to be compiled – Once you start to develop this function keep it unchecked. This will not prevent other developers from creating another function with the same name. After you complete development you can enable the checkbox.
Test function compilation – This button will help to test the function for any language errors. If errors, you will be notified.
Generate function – Only after doing the test compilation, you can click on the generate function button.
What happens on clicking the generate function button?
The function will be generated and ready to be used. You can find the source code generated in the server file system. Click on the generate function.
Go to your personal edition temp folder
C:PRPCPersonalEditiontempPRGenJavacompegarulesgenerated
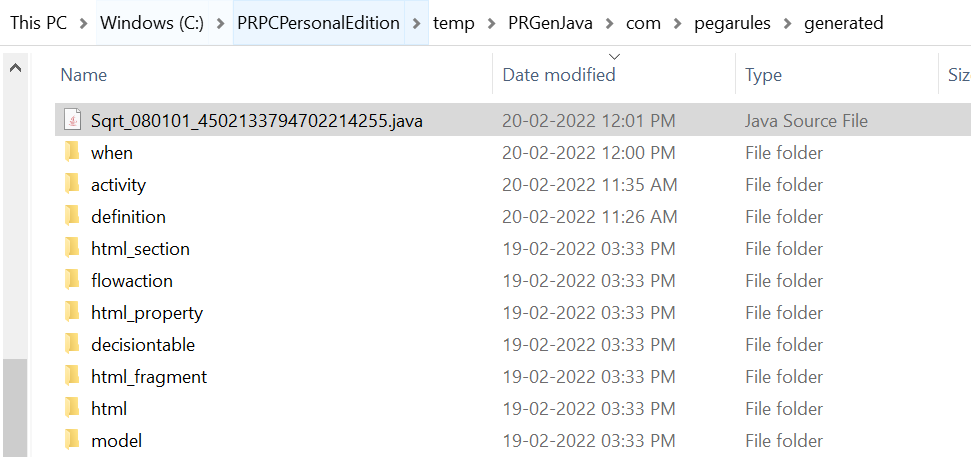
You see the Java source code is generated just now.
Generate Library – By clicking this button, all the functions that are part of the library will be generated. You need to handle this with extra care. Perform this only when you are sure all functions that are built are error free and ready to use.
Note: On multi-node system, the Pega system pulse will take care of syncing the functions compilation on all servers
What are the ways to generate functions and libraries in Pega?
Alert: The options here may vary based on your Pega versions.
1. You can use the generate library button in both the functions and library rule form to compile and generate functions. Make sure to click on generate library to make any changes to the function rule.
2. During import you can use compile library checkbox to compile the new library rules on import into a new environment
3. In the older versions you have a file in the temp folder – PegaRULES_Extract_Marker.txt file. This will delete all the generated Java files as well as the static content directory and some caches. Once the server is started, then all the libraries will be force-compiled.
Alias tab
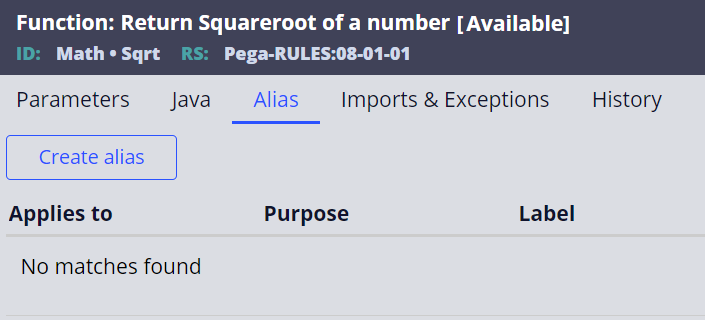
As you see function rule involves Java coding it may be difficult for everyone including the business users to understand its usage. Reports may use some standard functions to present the data in a desired format. In such case, users may be find it difficult to find and invoke the technical functions. To solve this, we have a separate rule type – function alias that provides a description or an easy way to refer to the functions.
Imports & Exceptions tab
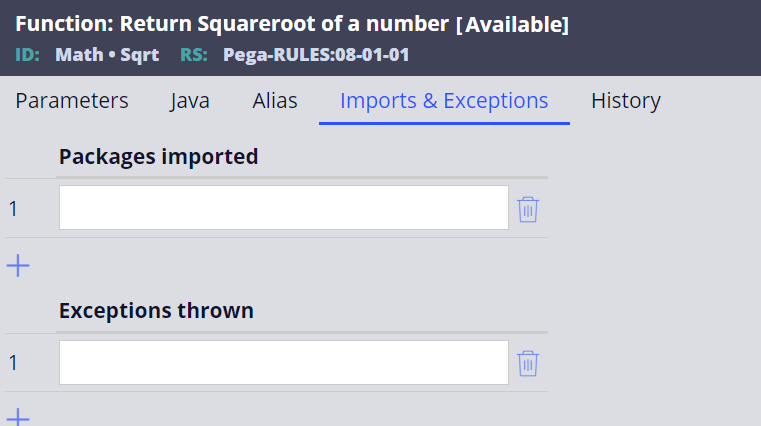
Normally java code in the functions rule by default can access the classes in the below packages
- Java util and Java lang packages
- Classes defined in the Pega Engine API
- Classes that are imported from the library rule form.
If you need to use any other classes apart from the above mentioned packages, then you can refer it in the packages imported array list.
If your function need to handle exceptions, you can also refer a fully qualified exception name in the exceptions thrown array list.
Till now we have seen the usage of OOTB library and function rule.
As a summary,
– Function is a block or reusable code that is intended to perform specific operations and a library is a collection of pre-written that includes the functions.
– Java API documentation defines all the packages, classes and functions that are inbuilt.
– Pega Engine API documentation defines all the Pega Java functions that are inbuilt in the Pega engine.
– Library and Function are two rule instances that can be configured to support standard Pega functions.
– Standard Pega functions can be referred to in the expression builder while the Pega Java function cannot be referred in the expression builder.
As a continuation in the next article, we will create a new library and function rule and see what are the ways function rule can be referenced.